Flex based Flickr API Authorization control
I just checked in some updates to the ActionScript 3 Flickr library. The biggest change is that I checked in a Flex control that will provide a UI and handle all of the communication to authorize an application with Flickr.
There is no documentation on it, and as I have built it for projects I am using, there are probably some API gaps, but it seems to be pretty solid.
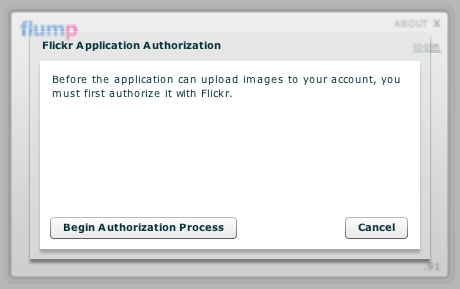
Here is a simple example of how to use it:
import com.adobe.webapis.flickr.authorization.events.AuthorizationEvent;
import com.adobe.webapis.flickr.authorization.AuthorizationView;
import mx.core.IFlexDisplayObject;
import mx.managers.PopUpManager;
//open the authorization panel
private function openAuthorization():void
{
var p:IFlexDisplayObject = PopUpManager.createPopUp(this, AuthorizationView, true);
var auth:AuthorizationView = AuthorizationView(p);
//get this from flickr
auth.flickrAPIKey = "XXXXXXXXXXXXXXXXXXXXX";
//get from flickr
auth.flickrAPISecret = "XXXXXXXXXXXXXXXXXXXX";
auth.isPopUp = false;
p.addEventListener(Event.CLOSE, onAuthorizationClose);
p.addEventListener(AuthorizationEvent.AUTHORIZATION_COMPLETE, onAuthorizationComplete);
PopUpManager.centerPopUp(p);
}
private function onAuthorizationClose(e:Event):void
{
PopUpManager.removePopUp(IFlexDisplayObject(e.target));
}
private function onAuthorizationComplete(e:AuthorizationEvent):void
{
trace(e.authToken);
trace(e.user.username);
}
Basically, this will open a panel that walks the user through the authorization steps (which requires a visit to the Flickr site in the user’s browser). Once the authorization is complete, an event is thrown with the authorization token (which should then be saved between app sessions).
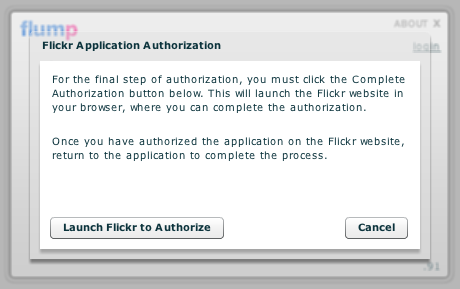
The api requires that your app have an api key and secret key, both of which you can grab from flickr at:
http://www.flickr.com/services/api/keys/apply/
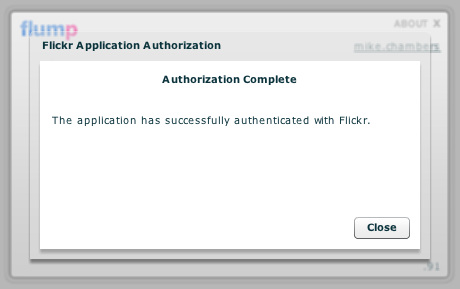
If you run into any issues, or have any suggestions either log a bug on the flickr lib project site, or post a comment here. Plus, if anyone thinks that can make the UI look prettier, just ping me.